Advanced Logging
Quokka provides live logging capabilities that allow you to see the values of variables and expressions, as well as runtime errors, directly in your editor.
The values and errors are displayed:
- inline in the editor, next to the code that produced them,
- in editor hover tooltips,
- in the Quokka output panel.
The values and errors are displayed:
- inlined in the editor, next to the code that produced them,
- in the Quokka output panel.
There are several ways to log values in Quokka:
console.log
statements,- identifier expressions (i.e. just typing a variable name),
- live comments (includes performance measurements),
console.log
statements,- identifier expressions (i.e. just typing a variable name),
- live comments (includes performance measurements),
- logpoints,
- value peek,
- automatic logging,
Show Line Value(s)
command.
console.log
and Identifier Expressions
You may use console.log
or identifier expressions (i.e. just typing a variable name) to log any values.
Note that when using identifier expressions for logging (for example, typing a
to see the value of the a
variable),
you may hit some limits in terms of the number of displayed properties and the logged object traversal depth. In this
case, you may use console.log(a)
to display objects without the limitations.
Logpoints Pro Feature
Logpoints allow editor breakpoints to be used to display the value of any expression. Using breakpoints to manage displaying values offers a number of advantages:
- No modification to source code is necessary
They offer a clear visual indicator of what is being logged (especially when placed within lines as
inline breakpoints
)- They are easily added and removed using familiar keyboard shortcuts (
F9
, or⇧ F9
for inline breakpoints) - No debugger required; zero setup / configuration and works seamlessly using Quokka’s runtime
- They are managed by your editor, and will persist when files are closed and reopened
- They can be added to functions and classes to log all lines within them
- No modification to source code is necessary
They offer a clear visual indicator of what is being logged (especially when placed within lines as
inline breakpoints
)They are easily added and removed using familiar keyboard shortcuts (usually
⌘F8
orF9
, depending on keybindings)- No debugger required; zero setup / configuration and works seamlessly using Quokka’s runtime
- They are managed by your editor, and will persist when files are closed and reopened
- They can be added to functions and classes to log all lines within them
Value Peek Pro Feature
Value Peek provides another way to quickly inspect values without modifying your code. When enabled, hovering over any
expression or selection will evaluate, capture, and display the associated value(s). To avoid clutter, in-editor display
of values is suppressed by default, but can be shown by using the Explore Value
icon (to the right of the value in
the hover).
Live Comments Pro Feature
Live Comments allow special comments to be used to display values. Inserting the special comment /*?*/
after an
expression (or just //?
after a statement) will log just the value of that expression. Like Logpoints,
Live Comments allow you to see the value right in the middle of an expression. For example, given a chain of
a.b().c().d()
, you may want to inspect the result of a.b().c()
before .d()
is called.
The example below shows how to log the runtime value of a.b().c()
:
a.b() .c() /*?*/ .d();
If you want to log the full expression of a.b().c().d()
then you can add a comment at the end of the expression:
a.b().c().d(); /*?*/// or justa.b().c().d(); //?
You may also write any JavaScript code right in the comment to shape the output. The code has the access to the $
variable which is the expression that the comment is appended to. The executed code is within a closure, so it also has
access to any objects that you may access from within the current lexical environment.
Note that there are no constraints in terms of what the comment code can do. For example, the watch comment below is
incrementing d.e
value, and returning $
, which points to the expression that the comment is appended to (a.b
).
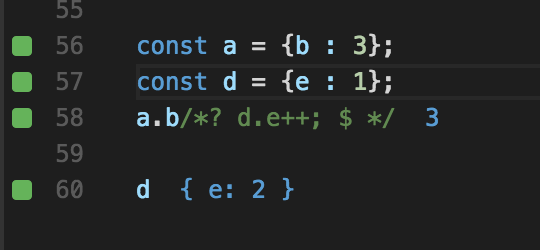
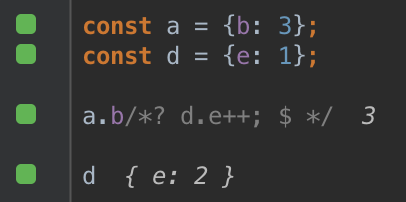
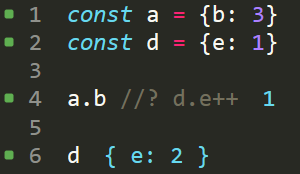
Also, unlike console logging, the special comment logging has some built-in smarts. For example, when you place the
comment after an expression that is a promise, the resolved value of the promise is logged. Similarly, if an
expression is an observable, then its values are displayed. So you don’t need to insert a .then
function to just
inspect the resolved value of the promise or a forEach
/subscribe
function to inspect the observable values.
Live performance testing
The feature allows to quickly see how various parts of your code perform. It can be very helpful for identifying possible bottlenecks in your app and for doing the performance optimization, or just for experimenting to see how different things perform.
Inserting the special comment /*?.*/
after any expression will report how much time it took to execute the
expression.
Adding the comment to an expression that gets executed multiple times, for example inside a loop, will make the tool to display total execution time, average execution time (total execution time divided by number of times the expression had been executed), minimum and maximum execution time.
You may also use the execution time reporting comment and add to it any expression that you may use in live comments to display both an expression execution time and execution result.
For example,
a() //?. $
displays a()
execution time and result.
Auto-Expand Comment
You may use Quokka’s auto-expand comment //?+
with small- to medium-sized objects when you want to expand all
properties (subject to limit described below). For example:
const obj = { a: { b: { c: { d: 1, }, }, },};
obj; //?+
Note that automatically expanded objects have the following limitations:
- Cyclic Depedencies are not automatically expanded
- Functions are not automatically expanded
- Strings beyond 8192 characters are not automatically expanded
- Only the first 100 properties on a single object will be expanded
- Only the first 100 elements of an array will be expanded
- Only the first 10 levels of nested properties will be expanded
- Only the first 5000 properties across all objects will be expanded
Live comment snippet
To save some time on typing the comment when you need it, you may create a code snippet with a custom keybinding.
To save some time on typing the comment when you need it, you may create a live template.
To save some time on typing the comment when you need it, you may create code snippets.
Automatic Logging Pro Feature
Show Value On Selection
If you want to quickly display an expression value, but don’t want to use logpoints or modify your code, you may do it by simply selecting an expression in an editor.
By default, Quokka will start with Show Value On Selection
disabled, but this can be changed with
configuration, and you may UI button to enable it just for the current file.
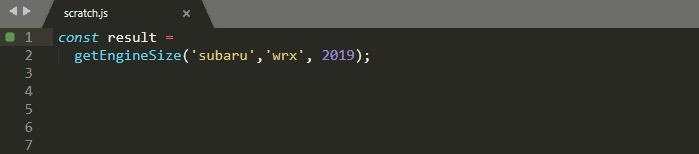
Automatically logged values are sticky which means that they survive your file changes while being updated as you type.
You may press Escape
to quickly clear a value or Escape Escape
to clear all values in a file, which are the
default keyboard shortcuts for Clear Value
and Clear File Values
commands accordingly.
You may press Escape
to clear a value or Escape Escape
to clear all values in a file. In addition to the keyboard
shortcuts there are also Clear value
and Clear file values
intention actions available.
Auto Log All Values
If you want Quokka to show the runtime values for every single line of your code then you may use the Auto Log
feature.
By default, Quokka will start with Auto Log
disabled, but this can be changed with
configuration, and you may use the Toggle Auto Log
command or UI button to enable
it just for the current file.
This feature is useful in scenarios such as when you are live coding and have intermediate results that you want to be displayed as you type.
Commands Pro Feature
The Logpoints and Live Comments features provide excellent ways to log expressions and
keep displaying values when you change your code, but they may not be suitable for all workflows. The Show Value
and
Show Line Values
commands can be used to quickly display values in the editor. Additionally, the Copy Value
command
can be used to directly copy an expression value.
Show Line Value(s)
If you want to output the values of multiple lines, you may use the Show Line Value(s)
command. This command will
output the same as Show Value
for the selected line(s) as if you had selected the entire line to display.
If you want to output the values of multiple lines, you may use the Show Line Value(s)
intention action. This action
will output the same as Show Value
for the selected line(s) as if you had selected the entire line to display.
Copy Value
If you want to copy an expression value to your clipboard without modifying your code, Live Value Display allows
you to do it with a special command (Copy Value
command, or with the Cmd + K, X
keyboard shortcut).
If you want to copy an expression value to your clipboard without modifying your code, Live Value Display allows
you to do it with a special intention action (Alt+Enter
& select the Copy Value
action).
Show Line Timing(s)
If you want to output the execution time of a line, you may use the Show Line Timing(s)
command. This command will
output the same as if you had used the //?.
comment for the selected line(s).
If you want to output the execution time of a line, you may use the Show Line Timing(s)
intention action. This
action will output the same as if you had used the //?.
comment for the selected line(s).